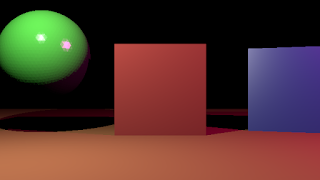
This is an article in a multipart series on the concepts of ray tracing. I am not sure where this will lead but I am open to suggestions. We will be creating code that will run inside Blender. Blender has ray tracing renderers of course but that is not the point: by reusing Python libraries and Blender's scene building capabilities we can concentrate on true ray tracing issues like shader models, lighting, etc.
I generally present stuff in a back-to-front manner: first an article with some (well commented) code and images of the results, then one or more articles discussing the concepts. The idea is that this encourages you to experiment and have a look at the code yourself before being introduced to theory. How well this works out we will see :-)
So far the series consists of the several articles labeled ray tracing concepts
I generally present stuff in a back-to-front manner: first an article with some (well commented) code and images of the results, then one or more articles discussing the concepts. The idea is that this encourages you to experiment and have a look at the code yourself before being introduced to theory. How well this works out we will see :-)
So far the series consists of the several articles labeled ray tracing concepts
Refactoring
To make it easier to caculate secondary rays like relected or refracted rays, we move the actual ray casting to its own function, which also makes the central loop that generates every pixel on the screen much more readable:for y in range(height): yscreen = ((y-(height/2))/height) * aspectratio for x in range(width): xscreen = (x-(width/2))/width # align the look_at direction dir = Vector((xscreen, yscreen, -1)) dir.rotate(rotation) dir = dir.normalized() buf[y,x,0:3] = single_ray(scene, origin, dir, lamps, depth)
Colored lights
Within thesingle_ray()
function we also add a small enhancement: now we calculate the available amount of light to use in reflections from the properties in the lamp data:
... for lamp in lamps: light = np.array(lamp.data.color * lamp.data.energy) ...
User interface
To allow the user to set the color and intensity ("energy") of the point lights we need the corresponding lamp properties panel (we only use color and energy):
This panel is added in the
register()
function:
from bl_ui import ( properties_render, properties_material, properties_data_lamp, ) ... identical code left out ... properties_data_lamp.DATA_PT_lamp.COMPAT_ENGINES.add(CustomRenderEngine.bl_idname)
No comments:
Post a Comment